jsAPI endpoints
waSurvey.getTasksByFormId
Description:
Gets a list of tasks for a specific form
Parameters:
Name | Type | Description |
---|---|---|
|
| The numeric id of the form. |
|
| The max number of records to return. |
|
| The offset of records for pagination. |
|
| A callback function which recieves an array of zero or more tasks. |
waSurvey.getTaskDefinition
Description:
Gets the task definition associated with a specific form
Parameters:
Name | Type | Description |
---|---|---|
|
| The numeric id of the form. |
|
| A callback function which recieves a task definition object. |
getServerUrl
Returns server URL.
WaSurvey.prototype.getServerUrl = function () {
return window.chrome.webview.hostObjects.sync._waSurvey.getServerUrl();
};
downloadAllFiles
Starts all attachments download process.
WaSurvey.prototype.downloadAllFiles = function () {
window._waSurvey.downloadAllFiles();
};
getPlatform
Returns platform name.
WaSurvey.prototype.getPlatform = function() {
return "iOS";
};
waSurvey.checkResponseExistence
Description:
Checks if a response with the specified offine respondent ID
Parameters:
Name | Type | Description |
---|---|---|
|
| The numeric value of the target form |
|
| The offline respondent id of the target response |
|
| Takes a boolean value indicating the existance of the response. |
updateResponse
Updates existing response with specified parameters in background.
Parameter formId: Int. Identifier of the form in which response should be updated.
Parameter responseId: String. Offline identifier of the response to be updated.
Parameter title: String. New title of the response.
Parameter results: JSON. An array of updated results. Follows the same structure for response results.
Parameter status: Int. New status of the response. One of the values form 'RESPONSE_STATUS'.
Parameter date: Double. UNIX Timestamp representing creation date of the response.
Parameter successCallback: function(String). Takes response's offline identifier as argument.
Parameter failureCallback: function(String). Takes error message as argument.
WaSurvey.prototype.updateResponse = function(formId, responseId, title, results, status, date, successCallback, failureCallback) {
var responseData = {
formId: formId,
responseId: responseId,
title: title,
results: results,
status: status,
date: date
};
SwiftWebViewBridge.callSwiftHandler(waSurvey.MESSAGE.UPDATE_RESPONSE, responseData, function(data) {
if (data.responseId != null) {
successCallback(data.responseId)
} else if (data.error != null) {
failureCallback(data.error)
} else {
failureCallback("Invalid format for 'updateResponse'.");
waSurvey.log("ERROR", "Invalid format for 'updateResponse'.");
}
});
};
createResponse
Creates response with specified parameters in background.
Parameter formId: Int. Identifier of the form in which response should be created.
Parameter title: String. Title of the response.
Parameter results: JSON. An array of results to be prefilled into response. Follows the same structure for response results.
Parameter status: Int. Status of the response. One of the values form 'RESPONSE_STATUS'.
Parameter date: Double. UNIX Timestamp representing creation date of the response.
Parameter successCallback: function(String). Takes response's offline identifier as argument.
Parameter failureCallback: function(String). Takes error message as argument.
WaSurvey.prototype.createResponse = function(formId, title, results, status, date, successCallback, failureCallback) {
var responseData = {
formId: formId,
title: title,
results: results,
status: status,
date: date
};
SwiftWebViewBridge.callSwiftHandler(waSurvey.MESSAGE.CREATE_RESPONSE, responseData, function(data) {
if (data.responseId != null) {
successCallback(data.responseId)
} else if (data.error != null) {
failureCallback(data.error)
} else {
failureCallback("Invalid format for 'createResponse'.");
waSurvey.log("ERROR", "Invalid format for 'createResponse'.");
}
});
};
requestExternalResources
Requests list of resources URI's for specified form.
Parameter formId: Int
Parameter successCallback: function([String]). Takes list of absolute urls for form resources.
Parameter failureCallback: function(String). Takes either "FORM_SYNCING" or "NO_FORM". "FORM_SYNCING" - Form is synchronizing at this moment. "NO_FORM" - Form is missing on device.
WaSurvey.prototype.requestExternalResources = function(formId, successCallback, failureCallback) {
var form = {
formId: formId
};
SwiftWebViewBridge.callSwiftHandler(waSurvey.MESSAGE.EXTERNAL_RESOURCES, form, function (data) {
if (data.resources != null) {
successCallback(data.resources)
} else if (data.error != null) {
failureCallback(data.error)
} else {
failureCallback("NO_FORM");
waSurvey.log("ERROR", "Invalid format for 'requestExternalResources'.")
}
});
};
showDialog
Requests to show custom dialog with specified information.
Parameter title: String
Parameter message: String
Parameter buttons: JSON = {
kind: "POSITIVE" | "NEGATIVE" | "NEUTRAL",
text: String
}
Parameter callback: function(String). Takes `kind` of selected button as an argument.
WaSurvey.prototype.showDialog = function(title, message, buttons, callback) {
var data = {
title: title,
message: message,
buttons: buttons.buttons
};
SwiftWebViewBridge.callSwiftHandler(waSurvey.MESSAGE.SHOW_DIALOG, data, function (data) {
var jsonString = JSON.stringify(data);
var obj = JSON.parse(jsonString);
callback(obj.kind);
});
};
setAutosaveOption
Toggles autosave option.
Param "isEnabled" - flag defining whether automatic save should be enabled.
WaSurvey.prototype.setAutosaveOption = function(isEnabled) {
this.isAutoSaveEnabled = isEnabled;
var data = {
isAutoSaveEnabled: isEnabled
};
SwiftWebViewBridge.callSwiftHandler(waSurvey.MESSAGE.AUTOSAVE_TRIGGER, data, null);
};
getAutosaveOption
Requests autosave option.
WaSurvey.prototype.getAutosaveOption = function() {
return this.isAutoSaveEnabled;
};
Log
Requests to log some data.
Param "severity" - log severity as int representation.
Severity can be defined either using integer (starting from 1.9.0) or string (for any version) values
Level - Int - String
Verbose - 2 - VERBOSE
Debug -3 - DEBUG
Info - 4 - INFO
Warning - 5 - WARN
Error - 6 - ERROR
Param "message" - log message
WaSurvey.prototype.log = function(severity, message) {
var logMetadata = {
severity: severity,
message: message
};
SwiftWebViewBridge.callSwiftHandler(waSurvey.MESSAGE.LOG_MESSAGE, logMetadata, null);
};
openGallery
Requests to open gallery.
Param "configuration" - parameters for gallery setup:
{
"question": {
"id": long,
"label": String
},
"isEditable": boolean,
"position": int,
"answers": {
"id": long,
"label": String
}
}
or
"answers": [
{
"id": long,
"label": String
}
]
}
WaSurvey.prototype.openGallery = function(configuration) {
SwiftWebViewBridge.callSwiftHandler(waSurvey.MESSAGE.OPEN_GALLERY, configuration, null);
};
requestBarcodeScan
Requests to open camera for scanning a barcode. Returns String in successful callback as a result, otherwise - an error.
WaSurvey.prototype.requestBarcodeScan = function(success, failure) {
SwiftWebViewBridge.callSwiftHandler(waSurvey.MESSAGE.SCAN_BARCODE, null, function (data) {
var jsonString = JSON.stringify(data);
var obj = JSON.parse(jsonString);
var barcode = obj.barcode;
if (barcode != null) {
success(barcode);
} else {
failure(obj.error);
}
});
};
loadDataModel
Requests one specified row from data model.
Param "params" - request in json representation:
{
"id": Integer
}
Param "success" - callback to be called upon successful completion,
Param "failure" - callback to be called in case of failure
WaSurvey.prototype.loadDataModel = function(params, success, failure) {
SwiftWebViewBridge.callSwiftHandler(waSurvey.MESSAGE.LOAD_DATA_MODEL_ROW, params, function (data) {
var jsonString = JSON.stringify(data);
var result = JSON.parse(jsonString);
if (result != null) {
success(result);
} else {
failure(result);
}
});
};
loadDataModelsFinalSelect
Requests "items" from dataModel by specified filters.
Param "params" - request in json representation:
{
"indexStart": Integer,
"indexEnd": Integer,
"filters": {
"logic": String,
"nodes": [
{
"logic": String,
"nodes": [{...}]
"name": String,
"columnId": String,
"isComplex": Boolean,
"operator": String,
"value": Object
}
]
"name": String,
"columnId": String,
"isComplex": Boolean,
"operator": String,
"value": Object
}
}
Param "success" - callback to be called upon successful completion,
Param "failure" - callback to be called in case of failure
WaSurvey.prototype.loadDataModelsFinalSelect = function(params, success, failure) {
SwiftWebViewBridge.callSwiftHandler(waSurvey.MESSAGE.LOAD_DATA_MODEL, params, function (data) {
var jsonString = JSON.stringify(data);
var result = JSON.parse(jsonString);
if (result != null) {
success(result);
} else {
failure(result);
}
});
};
loadDataModelsFilters
Requests "filters" from dataModel which will be used in final select.
Param "params" - request in json representation
{
"indexStart": Integer,
"indexEnd": Integer,
"columnId": Integer,
"filters": {
"logic": String,
"nodes": [
{
"logic": String,
"nodes": [{...}]
"name": String,
"columnId": String,
"isComplex": Boolean,
"operator": String,
"value": Object
}
]
"name": String,
"columnId": String,
"isComplex": Boolean,
"operator": String,
"value": Object
}
}
Param "success" - callback to be called upon successful completion,
Param "failure" - callback to be called in case of failure
WaSurvey.prototype.loadDataModelsFilters = function(params, success, failure) {
SwiftWebViewBridge.callSwiftHandler(waSurvey.MESSAGE.FILTER_DATA_MODEL, params, function (data) {
var jsonString = JSON.stringify(data);
var result = JSON.parse(jsonString);
if (result != null) {
success(result);
} else {
failure(result);
}
});
};
deleteFile
Requests to delete attached file.
Param "questionId" - question ID,
Param "answerId" - answer ID,
Param "shouldConfirmDeletion" - is action require confirmation
WaSurvey.prototype.deleteFile = function(questionId, answerId, shouldConfirmDeletion) {
var deletionMetadata = {
attachment: {
questionId: questionId,
answerId: answerId
},
shouldConfirmDeletion: typeof shouldConfirmDeletion === "undefined" ? true : shouldConfirmDeletion
};
SwiftWebViewBridge.callSwiftHandler(waSurvey.MESSAGE.DELETE_ATTACHMENT, deletionMetadata, function (data) {
var deletedAttachments = [
{
questionId: deletionMetadata.attachment.questionId,
answerId: deletionMetadata.attachment.answerId,
status: waSurvey.FILE_UPLOAD_STATUS.EMPTY
}
];
waSurvey._native2JS_notifyFilesChanged(deletedAttachments);
});
};
downloadFileCancel
Cancels download request.
WaSurvey.prototype.downloadFileCancel = function(questionId, answerId) {
var attachmentMetadata = {
questionId: questionId,
answerId: answerId
};
SwiftWebViewBridge.callSwiftHandler(waSurvey.MESSAGE.CANCEL_DOWNLOAD_ATTACHMENT,
attachmentMetadata,
waSurvey._native2JS_notifyFilesChanged)
};
downloadFile
Requests to download specified attach file from server.
Params "questionId" - question ID,
Param "answerId" - answer ID
WaSurvey.prototype.downloadFile = function(questionId, answerId) {
var attachmentMetadata = {
questionId: questionId,
answerId: answerId
};
SwiftWebViewBridge.callSwiftHandler(waSurvey.MESSAGE.DOWNLOAD_ATTACHMENT,
attachmentMetadata,
waSurvey._native2JS_notifyFilesChanged)
};
attachFile
Requests to attach file from device.
Param "sourceType" - source type (supported: "CAMERA" / "GALLERY"),
Param "questionId" - question ID,
Param "answerId" - answer ID,
Param "options":
{
"allType": boolean,
"imageEnable": boolean,
"imageFileSize": int,
"imageQuality": int,
"docEnable": boolean,
"docSize": long,
"otherEnable": boolean,
"fileSize": long,
"fileExtensions": String
}
WaSurvey.prototype.attachFile = function(sourceType, questionId, answerId, options) {
var configuration = {
sourceType: sourceType,
questionId: questionId,
answerId: answerId,
options: options
};
SwiftWebViewBridge.callSwiftHandler(waSurvey.MESSAGE.ATTACH_FILE, configuration, function (data) {
var jsonString = JSON.stringify(data);
var obj = JSON.parse(jsonString);
var files = obj.files;
waSurvey._native2JS_notifyFilesChanged(files);
});
};
removeFileChangeListener
Removes listening of file changes.
WaSurvey.prototype.removeFileChangeListener = function() {
this.attachCallBack = null;
this.removeFileChangeListener();
};
addFileChangeListener
Adds change listener for all attachments described in "attachmentsMetadata" parameter.
WaSurvey.prototype.addFileChangeListener = function(attachmentsMetadata, callBack) {
this.attachCallBack = callBack;
if (!callBack) {
return
}
this.listenersAboutFiles.push(callBack);
SwiftWebViewBridge.callSwiftHandler(waSurvey.MESSAGE.GET_ATTACHMENTS, attachmentsMetadata, function(data) {
waSurvey._native2JS_notifyFilesChanged(data);
});
};
goToVoting
Closes current form and opens another for specified response.
formId: Int. Identifier of the form in which response should be opened.
responseId: String?. Offline identifier of the response to be opened if provided. If nil received then a new response should be created for specified form.
pageIndex: Int. Index of page at which response should be opened.
isViewMode: Bool. Flag defining whether response should be opened in view mode.
query: String?. Parameters to be passed with page url for response that requires opening.
failureCallback: function(String). Takes either "FORM_SYNCING" or "NO_FORM". "FORM_SYNCING" - Form is synchronizing at this moment. "NO_FORM" - Form is missing on device.
WaSurvey.prototype.goToVoting = function(formId, pageIndex, responseId, isViewMode, query, failureCallback) {
var responseData = {
formId: formId,
responseId: responseId,
pageIndex: pageIndex > 0 ? pageIndex : 1,
mode: isViewMode ? waSurvey.MODES.VIEW : waSurvey.MODES.EDIT,
query: query
};
SwiftWebViewBridge.callSwiftHandler(waSurvey.MESSAGE.OPEN_RESPONSE, responseData, function(data) {
if (data == null) {
return
}
if (data.error != null) {
failureCallback(data.error)
} else {
failureCallback("Invalid format for 'goToVoting'.");
waSurvey.log("ERROR", "Invalid format for 'goToVoting'.");
}
});
};
saveChanges
Saves unsaved changes, updates response title and triggers callback.
Param "callback": callback to be triggered upon save completion.
WaSurvey.prototype.saveChanges = function(callback) {
var responseData = {
id: this.responseId,
offlineId: this.responseOfflineId,
results: this.results,
trace: JSON.parse(this.traces),
label: this.label,
status: this.status,
state: this.state
};
SwiftWebViewBridge.callSwiftHandler(waSurvey.MESSAGE.SAVE_RESPONSE_DATA, responseData, function (callbackData) {
callback();
if (responseData.status == waSurvey.RESPONSE_STATUS.COMPLETED || responseData.state == waSurvey.STATES.CLOSING) {
waSurvey.close();
}
});
};
getResponse
Returns response identifiers for saved responses.
When response hasn't been saved yet (e.g. Voting opened via "Add new response" action) this method won't return any identifiers.
You SHOULD NOT rely on absence of identifiers to determine when response is saved.
Use waSurvey.getStatus() to determine status of the response. When it is NEW - the response wasn't saved.
WaSurvey.prototype.getResponse = function() {
var responseData = {
"responseId": (typeof this.responseId === "undefined") || (this.status == waSurvey.RESPONSE_STATUS.NEW) ? null : this.responseId,
"responseOfflineId": (typeof this.responseOfflineId === "undefined") || (this.status == waSurvey.RESPONSE_STATUS.NEW) ? null : this.responseOfflineId
};
return responseData;
};
updateTask
Saves current task.
WaSurvey.prototype.updateTask = function(updatedTaskData) {
SwiftWebViewBridge.callSwiftHandler(waSurvey.MESSAGE.SAVE_TASK_DATA, updatedTaskData, null);
};
getTask
Returns current task.
WaSurvey.prototype.getTask = function() {
return waSurvey.task;
};
markAsChanged
Notifies form that response has unsaved changes. This causes Voting to present "Save Changes" dialog when user tries to close the Voting without tapping Save/Submit.
Note: This method has effect only in Edit Mode.
WaSurvey.prototype.markAsChanged = function() {
SwiftWebViewBridge.callSwiftHandler(waSurvey.MESSAGE.RESPONSE_CHANGED, null, null)
};
getMode
Returns current form mode.
Returns: Either of the following ["view"|"edit"].
WaSurvey.prototype.getMode = function() {
return this.mode;
};
Redirect
Redirects to specified form page.
Parameter pageIndex: Index of the page to be opened. (1-based).
WaSurvey.prototype.redirect = function(pageIndex) {
var data = {
pageIndex: pageIndex
};
SwiftWebViewBridge.callSwiftHandler(waSurvey.MESSAGE.PAGE_REDIRECT, data, null);
};
setState
Sets waSurvey's state property.
Parameter state: A new state to which Voting should be moved.
Parameter state values
this.STATES = {
LOADING: "loading",
OPEN: "open",
CLOSING: "closing",
CLOSED: "closed"
};
WaSurvey.prototype.setState(state)
getState
Returns current state of the form page, can be one of the following states:
Return Value
this.STATES = {
LOADING: "loading",
OPEN: "open",
CLOSING: "closing",
CLOSED: "closed"
};
WaSurvey.prototype.getState()
getRespondentResults
Returns results of specified response. If response or form doesn’t exist returns error.
WaSurvey.prototype.getRespondentResults(formId, offlineRespondentId, successCallback, failureCallback)
formId - id of the Form
offlineRespondentId - response offline id
successCallback - callback for passing successful result
failureCallback - callback for passing error
To notify success callback uses:
"javascript:waSurvey._notifySuccess($result, $successCallbackId, $failureCallbackId);"
To notify failure callback uses:
"javascript:waSurvey._notifyFailure(\"$errorMessage\", $successCallbackId, $failureCallbackId);"
vpFindAnswer("QX.AY")
Returns an object of an AnswerHandler type that corresponds to the parameter.
CODE
|
vpFindColumn("QX.AY.CZ")
Returns an object of a ColumnHandler type that corresponds to the parameter.
CODE
|
vpFindElement("QX.AY.CZ")
Returns an object of an ElementHandler type that corresponds to the parameter.
CODE
|
vpFindQuestion("QX")
Returns an object of a QuestionHandler type that corresponds to the parameter.
CODE
|
vpGetAllAnswerRateDifferent
pGetAllAnswerRateDifferent(vpGetAllQuestionRateDifferent()[0]) - returns an array of answer objects for the 'Rate different items along the scale of your choice' question object passed as it’s parameter.
For example, if the question has 3 answer options, you will get the following result:

vpGetAllQuestionRateDifferent
vpGetAllQuestionRateDifferent() function returns an array with data for all 'Rate different items along the scale of your choice' questions that you have on the page:
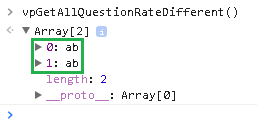
vpGetAllQuestionRateDifferent()[0] returns an array with data for particular question. [0] for Q1, [1] for Q2, etc. The numbering of elements starts from 0:

vpGetAnswersByQuestionId(id)
Returns an array of AnswerHandler elements that belong to a specific question
CODE
|
vpGetElements(String reference);
This function returns an array containing 1 DOM object that corresponds to the form inputs of question, answer option or a column. Examples:
vpGetElements("Qx")[0]; - a text area element of a multiline question;
vpGetElements("Qx.Ay")[0]; - returns a DOM object corresponding to an input of the answer option. Applicable to all questions, except Multiline, 3D matrix and Rate different.
vpGetElements("Qx.Ay.Cz")[0]; - for 3dMatrix and Rate Different only - returns inputs of a column
vpGetElements("Qx.Ay.Az")[0]; - for Compare one by one only - returns input of an answer cell.
vpGetElementsByQuestionId(id)
Returns an array of ElementHandler elements that belong to a specific question.
CODE
|
vpGetImage(String reference);
Examples:
vpGetImage("Qx");- returns an image DOM object attached to a question
vpGetImage("Qx.Ay"); - returns an image DOM object attached to an answer option;
vpGetLabel(String reference);
This function returns a DOM object of a cell that contains a label for question, answer option or column. Examples:
vpGetLabel("Qx");
vpGetLabel("Qx.Ay");
vpGetLabel("Qx.Cy");
vpGetQuestionByQuestionId(id)
Returns a QuestionHandler element for a question with certain Id
function vpGetQuestionByQuestionId(id)
{
return mySurvey.getQuestionByQuestionId(id);
}
vpGetResults
The function returns an array of JavaScript objects containing information about answers provided for a specific question:
Example Usage:
vpGetResults("Qx"); - returns an array of answer objects provided for a specific question.
vpGetResults("Qx.Ay"); - returns an array of answer objects provided for a specific answer.
vpGetResults("Qx.Ay.Cz"); - returns an array of answer objects provided for a specific answer-column.
This function allows to get the URL of a file attached to response. To get the URL of a file attached via Online voting (e.g. via browser), specify the question and answer option, number of the element and add urlDownload field. For instance, this function
CODE
|
will return the URL of a file attached to the first answer option of the second question of your form/survey via Online voting.
To get the URL of a file attached via Offline voting (e.g. via mobile application or Windows desktop application), specify the question and answer option, number of the element and add getUrlDownload field. For instance, this function
CODE
|
will return the URL of a file attached to the first answer option of the third question of your form/survey via Offline voting.
Answer object example:
CODE
|
You can also request the content of the FILE UPLOAD question by adding the getFileContent field via voting.
vpGetResults("Qx.Ay")[0].getFileContent().then(function(content){console.log("File content: " + content)},function(error){console.log("Error: "+error)});
vpGetStructure
The function returns a JavaScript object containing information about the survey/form structure.
Example Usage:
vpGetStructure();
Result:
{surveyId: XXXXXXX, questionsMap: {…}, questionsSorted: Array(1), questionsByVP: {…}, surveyHeader: "", …}
vpHide(String reference, boolean hide);
Examples:
vpHide("Qx", <true,false>); - shows or hides entire question block
vpHide("Qx.Ay", <true,false>); - shows or hides an answer option
vpHide("Qx.Ay.Cz", <true,false>); - shows or hides a cell of a 3D matrix or a Rate along scale
vpHide("Qx.Cz", <true,false>); - shows or hides the entire column of a 3D matrix or Rate along scale
vpHide("Qx.Ay.Az", <true,false>); - shows or hides an option of a question of Compare one against another type. reference is a reference to a survey element
hide - if set to true hides a selected element. If set to false - show it.
vpResetResults
The function reset results for a specific question/answer/column.
Example Usage:
vpResetResults("Qx"); - clears results for the whole question.
vpResetResults("Qx.Ay"); - clears results for a specified answer.
vpResetResults("Qx.Ay.Cz"); - clears results for a specified answer-column.
vpResetResults("Qx.Cz"); - clears results for a specifies column.
vpSetResults
The function sets an answer to a selected state / sets an answer field value to a specifies string.
Example Usage:
vpSetResults("Qx.Ay"); - sets an answer to 'selected' state. Applicable for 'fixed answer' question/answer types.
vpSetResults("Qx.Ay.Cz"); - sets an answer-column to 'selected' state. Applicable for 'fixed answer' question/answer types.
vpSetResults("Qx.Ay", "some String value here"); - sets an answer to a specified value. Applicable for 'open-ended' question/answer types.
vpSetResults("Qx.Ay.Cz", "some String value here"); - sets an answer-column to a specified value. Applicable for 'open-ended' question/answer types.
vpSetResults("Qx.Ay", "test content", {"fileName":"1.txt"}).then(function(success){console.log("Success: "+success)},function(error){console.log("Error: "+error)}); - sets a file attachment to the FILE UPLOAD question.
window.ksAPI.recalculateCalcValues()
ksAPI.recalculateCalcValues allows to create Calculated value based on previously created Calculated value. For example, if numeric calculation value was set for A4 of the Q2 as a multiplication of Q1.A1 and Q1.A2 values, it is possible to use Q2.A4 question reference in the formula for calculated value on Q3, and system will use the result of Q1.A1 and Q1.A2 values multiplication. This means, that this function re-calculates Calculated values each time when it us used for respective answer option and allows to simplify the process of Calculated values formulas creation.
CODE
|
where
RESULTS_SCORES is a Question identifier of the question the Calculated value is applied to.
vpGetIdentifiersMap()
Returns a list of identifiers and corresponding to them question references Qx.Ay.
For instance, if you have a form with one question and one answer option, e.g. Q1.A1, and you applied question and answer identifiers(Question_id and Answer_id respectively), this method will return the following object:
{Answer_id: "Q1.A1", Question_id: "Q1"}
vpGetIdentifierByReference()
Returns a list of identifiers by corresponding to them question references Qx.Ay.
For instance, if you have a form with one question and one answer option, e.g. Q1.A1, and you applied question and answer identifiers(Question_id and Answer_id respectively), this method will return the following objects(depending on parameters):
vpGetIdentifierByReference()
undefinedvpGetIdentifierByReference("Q1")
"Question_id"vpGetIdentifierByReference("Q1.A1")
"Answer_id"
vpGetReferenceByIdentifier()
Returns a list of question references by corresponding to them question and answer identifiers
For instance, if you have a form with one question and one answer option, e.g. Q1.A1, and you applied question and answer identifiers(Question_id and Answer_id respectively), this method will return the following objects(depending on parameters):
vpGetReferenceByIdentifier()
undefinedvpGetReferenceByIdentifier("Question_id")
"Q1"vpGetReferenceByIdentifier("Answer_id")
"Q1.A1"
vpIsViewMode()
This function returns a Boolean object (true or false response) indicating whether the Form/Survey is opened in Report-by-Respondent mode.
vpIsOfflinePDFExport()
This function returns a Boolean object (true or false response) indicating whether the Form/RBR is opened in PDF export mode in offline mobile applications.
vpGetKeyFields()
vpGetKeyFields("Qx") - returns a result of the key field(s) in Object Lookup or Multiple Object Lookup questions. In Qx reference, x is the number of the question, for which the result should be returned. This function will return an empty array [] if the question is not answered.
The result may look like this:
CODE
|
window.ksAPI.runCustomCode
This method is used to run custom code on voting page after the page is loaded and voting results are restored on page. For example, inside this function you can access voting page elements or voting results using other JS API functions like vpFindQuestion or vpGetResults.
Usage:
CODE
|
The code executed on each page. It runs on the voting page as soon as on the RBR page. Also it is called in the browser, offline cordova app and native app.
There is no need to wrap window.ksAPI.runCustomCode in the "ready" function since under the hood the custom code is executed inside "ready" function.
prepareForExport
/**
@param {Function} callback - function to execute.
@param {Number} timeout - calls a callback function after a number of milliseconds.
*/
var prepareForExport = function (callback, timeout);
This function executes primary functions before generating a PDF file with voting results available on a mobile device. It awaits completion of all executing and registered (or existing) functions in the array "customScriptCallbacksForExport". Since the callback function is the first argument of the function, the callback function will be executed after all functions are executed.
Before the Callback function execution, the prepareForExport function does the following:
Removes Back, Next, Submit and Save buttons on a voting page;
Removes the Autosave block;
Replaces a picture from an answer option of the File Upload question type in case there is no picture on a device but on the server;
Removes action buttons from an answer option of the File Upload question type above a picture;
Removes a block with links to other pages;
Removes a block with links to other pages.
Description of arguments of function:
Callback: a function that is responsible for printing pages in PDF format.
Timeout: an argument of a function (not mandatory) that could be used for the Callback function execution.
runAfterActions
/**
@param {Function} code - function to execute.
*/
var runAfterActions = function (code);
The function executes the Callback function, which includes the 'code' argument. The execution is guaranteed only after Set Value, Show/Hide, and Calculated Value actions are performed.
ksAPI.vpShowLoader
The following methods, ksAPI.vpShowLoader, ksAPI.vpHideLoader, and ksAPI.vpKeepLoaderAfterLoad, are used to manage the visibility of the animation, shown until custom JS code is ready to start voting, and its compatibility with custom spinners.
The vpKeepLoaderAfterLoad with true/false parameters is used to ignore calls to the vpHideLoader method.
These methods should be added to the form’s script as raw(inline) JS code, but not inside other functions like runAfterActions or similar.
Example:
ksAPI.vpKeepLoaderAfterLoad(true);
ksAPI.runCustomCode( function() {
myDefferedAction().then( function() { ksAPI.vpHideLoader(); } )
});
vpCompleteRespondent
/**
@param {Number} respondentId - Identificator of respondent.
*/
var vpCompleteRespondent = function (respondentId);
The function calls the server code to change a respondent's form status. For instance, it can change the In Progress status to Completed. Used for forms which are launched to a Contact manager or Task Definition.
window.ksAPI.runBeforeSetValue
ksAPI.runBeforeSetValue can be executed before the Set Value feature processing. This function accepts a custom function as its parameter, which allows to block execution of the Set Values feature when a form is loading.
If you pipe some values from one form to another and don't want certain Set Values to overwrite the values that you pipe, now you can block the execution of such Set Values.
If the value in a Set Value condition field changes, the Set Value expression will be triggered.
If the same question (e.g., Q1) has several Set/Clear Value expressions configured to set/clear its value, referencing Q1 in the Custom Function will block execution of all the Set/Clear Value expressions configured for such question.
Examples:
Examples
Blocking all the Set Value expressions on form load
CODE
|
Blocking certain Set Value expressions via question reference
CODE
|
Blocking certain Set Value expressions via Answer option reference
CODE
|
Blocking certain Set Value expressions via column reference (for the Rate different items and 3D matrix question types)
CODE
|
vpGetDataModelValues
The function allows getting the CM/DM values for the Portal user.
Input parameters:
humanExpression - string, Lookup question reference
options - object:
columns - array of column names that should be returned
limit - int, number that limits (not more than) amount of records that should be returned by the function
offset - int, number of records that should be skipped
error - function, error callback
success - function, success callback
Output:
object:
items - array of data model objects (key-value pairs, key is field id, value is value of the field in the DM object)
total - number, that shows total number of objects in the requested data model
Examples of usage:
Successful call with limit and offset
CODE
|
output in console:
CODE
|
Invalid question
CODE
|
output in console:
CODE
|
With columns filter and callbacks for success and error
CODE
|
output in console:
CODE
|
Columns could be specified by their ids or by names, f.e. ["XLOGIN", "XEMAIL"] if real column names in CM are "Login", "Email".
If the column name contains a space, it should be replaced by IA, f.e. [“XMIDDLEIANAME”] for “Middle name”.
With results filter
Complex expressions, using any combination of "AND" and "OR" logic, are allowed to combine multiple filtering conditions (e.g., to filter by date range, etc.).
Responses can be filtered by “DATE”, “STRING”, and “DECIMAL” data types.
The following filters are supported:
LESS_THAN
LESS_OR_EQUAL_THAN
GREATER_THAN
GREATER_OR_EQUAL_THAN
EQUALS
DOES_NOT_EQUAL
CONTAINS
DOES_NOT_CONTAIN
{
"offset": 0,
"limit": 100,
"filter": {
"type" : "LOGIC",
"operator" : "OR",
"subNodes" : [ {
"type" : "STRING",
"fieldId" : 63,
"operator" : "EQUALS",
"value" : "David"
}, {
"type" : "DATE",
"fieldId" : 65,
"operator" : "EQUALS",
"value" : "2006-06-26T00:00:00+03:00"
}, {
"type" : "LOGIC",
"operator" : "AND",
"subNodes" : [ {
"type" : "STRING",
"fieldId" : 63,
"operator" : "EQUALS",
"value" : "Jane"
}, {
"type" : "DECIMAL",
"fieldId" : 71,
"operator" : "EQUALS",
"value" : 32.0
}]
}]
}
}
output in console:
{items: Array(2), total: 7}
items: Array(2)
0: {75: 'user4@mail.com', 77: 'userX1'}
1: {75: 'user5@mail.com', 77: 'userX5'}
length: 2
[[Prototype]]: Array(0)
total: 7
[[Prototype]]: Object
vpAddEventHandler
The function returns unique string key of the added event. This key can be used for remove handler.
It returns null in case of wrong reference format or if handler is not a function.
CODE
|
Reference - reference to the answer. Can be QxAyCz or question/answer identifier.
CurrentResults and previousResults - the same object that is returned by vpGetResults(reference).
Initiator - can be CUSTOM_JS_ACTION if triggered by JSAPI function (like vpSetResult) or USER_ACTION if triggered by user input on page.
Example of usage
CODE
|
Execute vpSetResults("Q1.A1").
Result: There will be an alert with the message:
CODE
|
vpGetEventHandlers
This function returns an array of custom event handler objects.
CODE
|
It is possible to use the filter vpGetEventHandlers(filter), where the filter can be an object or undefined with the next structure
CODE
|
If the filter contains a reference, then all the related events will be returned including descendants.
For example, for 'Q1' - all the question's custom events will be returned even created like vpAddEventHandler("Q1.A1.C1").
If the filter is undefined or empty object then all the custom events will be returned.
vpRemoveEventHandlers
This function removes custom handlers by reference or by handler keys or removes all the custom handlers if the filter is undefined or empty object.
Returns array of removed custom event handler objects with the next structure:
{
originalReference,
key,
questionId,
answerId,
columnId,
originalEventHandler
}
It is possible to use the filter vpRemoveEventHandlers(filter), where the filter can be an object or undefined with the next structure
CODE
|
If the filter contains a reference, then all the related events will be removed including descendants.
For example for ‘Q1’ - events like Q1.A1, Q1.A1.C1 will be removed as well as Q1.
handlerKeys is useful to remove individual handlers, for example two handlers was added to the same reference
CODE
|
To remove only the first handler, but to leave the second one
CODE
|
It’s just a “CRUD” API for custom event handlers, it does not change existing events.
For example:
vpAddEventHandler('Q1', handler1);
vpRemoveEventHandlers({reference: 'Q1.A1'});
It does NOT mean that we “modify“ somehow existing handler1 and after remove it will be triggered for all the Q1 changes except Q1.A1
. It only means that we remove all the handlers with reference Q1.A1 or Q1.A1.C1 if they exist.
getUploadedFilesUrls( offlineResponseId, aOReferencesArray)
Allows to get the uploaded file URLs.
offlineResponseId - offline response Id assigned on offline device
AOReferense - array of strings with the references to AO, up to 1000 elements
Reference could be in the following format:
Qx.Ay
questionIdentifier.Ay
answerIdentifier
Returns promise
Example
getUploadedFilesUrls('45a5f61c-7025-47e3-8db8-e66c922cb8e7', ['Q1.A1'])
getUploadedFilesUrls('45a5f61c-7025-47e3-8db8-e66c922cb8e7', ['fu2_ao_1'])
getUploadedFilesUrls('45a5f61c-7025-47e3-8db8-e66c922cb8e7', ['fu3_q3.A2'])
Small piece of code to test in console:
getUploadedFilesUrls('45a5f61c-7025-47e3-8db8-e66c922cb8e7', ['Q1.A1']).then(result => http://console.info ('result: ', JSON.parse(result.msg)), fail => console.error('Failed: ', fail) );
ApiGW:
endpoint - /gw/v3/results/files/urls/
accepts the POST requests
Payload
{
"respondentOfflineId": "
{offlineRespondentId}
",
"answers":
{answerIdentifiersArray}
// up to 1000 elements
}
Example:
{ "respondentOfflineId": "45a5f61c-7025-47e3-8db8-e66c922cb8e7", "answers": [ "Q1.A1" ] }
vpIsAllowEditResponses()
This method checks resubmit behavior for portal or offline app form.
CODE
|
Returns:
For forms not launched to Contact Manager and not used in Task Definition:
always true
For forms launched to Contact Manager:
false - when
"Edit completed" is unchecked on Publish to Contact Manager page
"Do not allow respondent back into survey" is checked on Form Settings page ("Allow only one response per form" is also checked on Publish page)
true - in all other cases
For tasks: always true